编写自定义 Loader 和 Plugin 是 Webpack 高级用法的核心部分。以下是详细的步骤和示例,帮助你理解如何编写自定义 Loader 和 Plugin。
一、编写自定义 Loader
1. Loader 的基本结构
Loader 是一个函数,接收输入内容(通常是文件内容),并返回处理后的内容。Loader 可以是同步的,也可以是异步的。
同步 Loader 示例
module.exports = function(source) {
// source 是文件内容
const result = source.replace('World', 'Loader'); // 替换内容
return result; // 返回处理后的内容
};
异步 Loader 示例
module.exports = function(source) {
const callback = this.async(); // 获取异步回调函数
setTimeout(() => {
const result = source.replace('World', 'Loader');
callback(null, result); // 返回处理后的内容
}, 1000);
};
2. Loader 的配置
编写完 Loader 后,需要在 webpack.config.js
中配置。
示例配置
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js'
},
module: {
rules: [
{
test: /.txt$/, // 匹配 .txt 文件
use: [
{
loader: path.resolve(__dirname, 'loaders/my-loader.js') // 使用自定义 Loader
}
]
}
]
}
};
3. Loader 的常用 API
this.async()
:获取异步回调函数。this.callback(err, content, sourceMap?)
:返回处理结果,支持错误、内容和 sourceMap。this.resourcePath
:当前文件的路径。this.query
:获取 Loader 的配置参数。
二、编写自定义 Plugin
1. Plugin 的基本结构
Plugin 是一个类,必须实现 apply
方法。apply
方法接收 compiler
对象,通过监听 Webpack 的生命周期钩子来执行任务。
示例 Plugin
class MyPlugin {
apply(compiler) {
compiler.hooks.emit.tap('MyPlugin', (compilation) => {
console.log('MyPlugin is running!');
// 在打包完成后执行一些操作
for (const name in compilation.assets) {
if (name.endsWith('.js')) {
const contents = compilation.assets[name].source();
const withoutComments = contents.replace(//*[sS]*?*//g, '');
compilation.assets[name] = {
source: () => withoutComments,
size: () => withoutComments.length
};
}
}
});
}
}
module.exports = MyPlugin;
2. Plugin 的配置
编写完 Plugin 后,需要在 webpack.config.js
中配置。
示例配置
const MyPlugin = require('./plugins/my-plugin');
module.exports = {
entry: './src/index.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js'
},
plugins: [
new MyPlugin() // 使用自定义 Plugin
]
};
3. Plugin 的常用 API
compiler.hooks
:访问 Webpack 的生命周期钩子。compile
:编译开始时触发。emit
:生成资源到输出目录之前触发。done
:编译完成后触发。
compilation.assets
:访问当前编译的资源文件。compilation.addModule
:添加模块。
三、完整示例
1. 自定义 Loader 示例
Loader 文件:loaders/my-loader.js
module.exports = function(source) {
return source.replace('World', 'Loader');
};
Webpack 配置:webpack.config.js
const path = require('path');
module.exports = {
entry: './src/index.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js'
},
module: {
rules: [
{
test: /.txt$/,
use: [
{
loader: path.resolve(__dirname, 'loaders/my-loader.js')
}
]
}
]
}
};
2. 自定义 Plugin 示例
Plugin 文件:plugins/my-plugin.js
class MyPlugin {
apply(compiler) {
compiler.hooks.emit.tap('MyPlugin', (compilation) => {
console.log('MyPlugin is running!');
for (const name in compilation.assets) {
if (name.endsWith('.js')) {
const contents = compilation.assets[name].source();
const withoutComments = contents.replace(//*[sS]*?*//g, '');
compilation.assets[name] = {
source: () => withoutComments,
size: () => withoutComments.length
};
}
}
});
}
}
module.exports = MyPlugin;
Webpack 配置:webpack.config.js
const path = require('path');
const MyPlugin = require('./plugins/my-plugin');
module.exports = {
entry: './src/index.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js'
},
plugins: [
new MyPlugin()
]
};
四、总结
1. 自定义 Loader
- 作用:处理文件内容。
- 实现:导出一个函数,接收文件内容并返回处理后的内容。
- 配置:在
module.rules
中配置。
2. 自定义 Plugin
- 作用:扩展 Webpack 功能,操作整个构建过程。
- 实现:导出一个类,实现
apply
方法,监听 Webpack 生命周期钩子。 - 配置:在
plugins
中配置。
通过编写自定义 Loader 和 Plugin,可以灵活扩展 Webpack 的功能,满足特定项目的需求。
- - - - - - - 剩余部分未读 - - - - - - -
扫描关注微信公众号获取验证码,阅读全文
你也可以查看我的公众号文章,阅读全文
你还可以登录,阅读全文
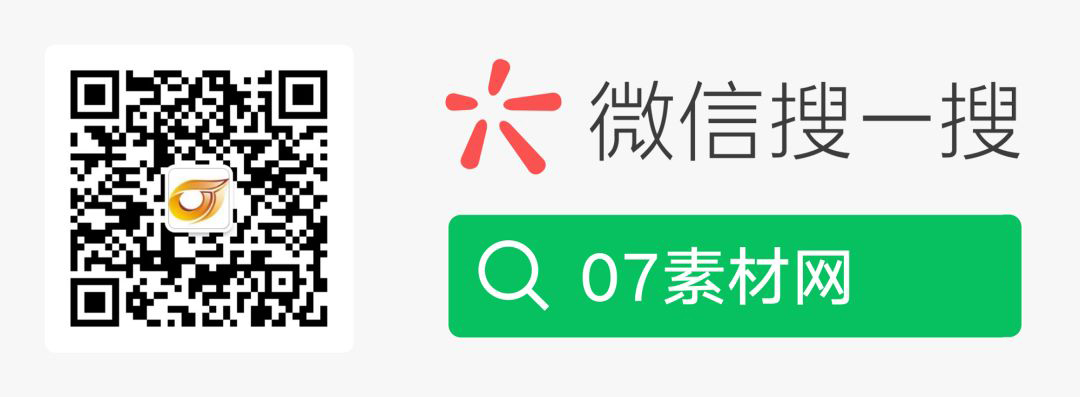
内容由AI生成仅供参考和学习交流,请勿使用于商业用途。
出处地址:http://www.07sucai.com/tech/658.html,如若转载请注明原文及出处。
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。