在 Node.js 项目中使用 TypeScript 可以提供更好的类型安全性和代码提示,帮助你编写更健壮和可维护的代码。以下是如何在 Node.js 中使用 TypeScript 的详细步骤和示例:
1. 初始化项目
首先,创建一个新的 Node.js 项目并初始化 package.json
文件。
mkdir my-node-app
cd my-node-app
npm init -y
2. 安装 TypeScript
在项目中安装 TypeScript 和 ts-node
。ts-node
是一个 TypeScript 执行环境,可以直接运行 TypeScript 文件。
npm install --save-dev typescript ts-node
3. 配置 TypeScript
在项目根目录下创建一个 tsconfig.json
文件,用于配置 TypeScript 编译选项。
{
"compilerOptions": {
"target": "ES6",
"module": "commonjs",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"outDir": "./dist"
},
"include": ["src/**/*"],
"exclude": ["node_modules"]
}
4. 创建项目结构
创建一个 src
目录来存放 TypeScript 源代码,并在其中创建一个 index.ts
文件。
mkdir src
touch src/index.ts
5. 编写 TypeScript 代码
在 src/index.ts
文件中编写一些简单的 TypeScript 代码。
// src/index.ts
import express, { Request, Response } from 'express';
const app = express();
const port = 3000;
app.get('/', (req: Request, res: Response) => {
res.send('Hello, world!');
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
6. 安装必要的依赖
安装 express
和 @types/express
,后者是 express
的类型定义文件。
npm install express
npm install --save-dev @types/express
7. 运行 TypeScript 代码
使用 ts-node
直接运行 TypeScript 文件。
npx ts-node src/index.ts
你应该会看到以下输出:
Server is running on http://localhost:3000
8. 编译 TypeScript 代码
如果你想将 TypeScript 代码编译为 JavaScript 代码,可以使用 tsc
命令。
npx tsc
编译后的 JavaScript 代码会输出到 dist
目录中。
9. 运行编译后的 JavaScript 代码
编译完成后,你可以使用 node
命令运行编译后的 JavaScript 代码。
node dist/index.js
10. 使用 nodemon
自动重启
为了在开发过程中自动重启服务器,你可以使用 nodemon
和 ts-node
。
首先,安装 nodemon
:
npm install --save-dev nodemon
然后,在 package.json
中添加一个启动脚本:
{
"scripts": {
"start": "nodemon --exec ts-node src/index.ts"
}
}
现在,你可以使用以下命令启动开发服务器:
npm start
nodemon
会监视文件变化,并在文件更改时自动重启服务器。
11. 使用 eslint
进行代码检查
为了保持代码风格一致,你可以使用 eslint
进行代码检查。
首先,安装 eslint
和相关插件:
npm install --save-dev eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin
然后,初始化 eslint
配置:
npx eslint --init
在初始化过程中,选择以下选项:
- How would you like to use ESLint? To check syntax, find problems, and enforce code style
- What type of modules does your project use? JavaScript modules (import/export)
- Which framework does your project use? None of these
- Does your project use TypeScript? Yes
- Where does your code run? Node
- How would you like to define a style for your project? Use a popular style guide
- Which style guide do you want to follow? Airbnb
- What format do you want your config file to be in? JSON
初始化完成后,eslint
会生成一个 .eslintrc.json
文件。你可以根据需要调整配置。
最后,在 package.json
中添加一个 lint
脚本:
{
"scripts": {
"lint": "eslint src/**/*.ts"
}
}
现在,你可以使用以下命令进行代码检查:
npm run lint
12. 使用 prettier
进行代码格式化
为了保持代码格式一致,你可以使用 prettier
进行代码格式化。
首先,安装 prettier
:
npm install --save-dev prettier eslint-config-prettier eslint-plugin-prettier
然后,在 .eslintrc.json
中添加 prettier
插件:
{
"extends": [
"airbnb-base",
"plugin:@typescript-eslint/recommended",
"plugin:prettier/recommended"
],
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint", "prettier"],
"rules": {
"prettier/prettier": "error"
}
}
最后,在 package.json
中添加一个 format
脚本:
{
"scripts": {
"format": "prettier --write src/**/*.ts"
}
}
现在,你可以使用以下命令进行代码格式化:
npm run format
总结
在 Node.js 中使用 TypeScript 可以提供更好的类型安全性和代码提示。你可以使用 ts-node
直接运行 TypeScript 代码,使用 tsc
编译 TypeScript 代码,使用 nodemon
自动重启服务器,使用 eslint
进行代码检查,以及使用 prettier
进行代码格式化。理解这些工具的使用场景和语法,可以帮助你编写出更健壮和可维护的 Node.js 代码。
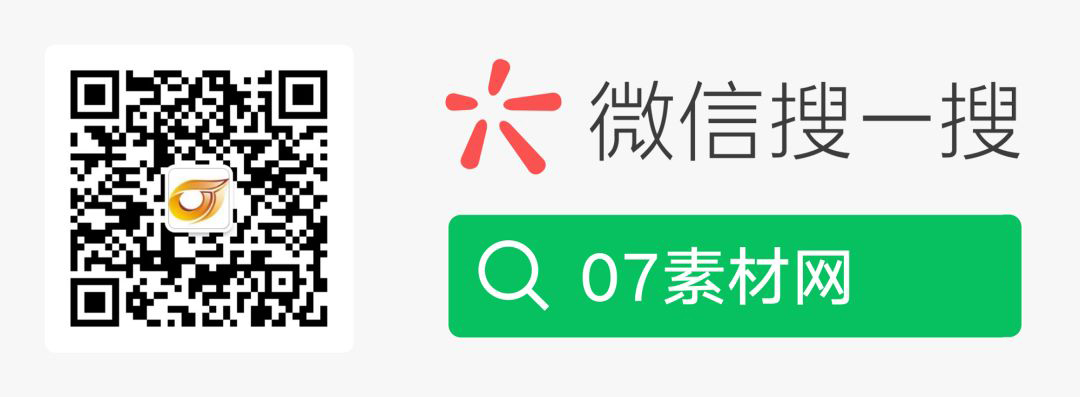